SyncedKey Class
Alternative way of implementing InputSynchronizable.
Namespace: AlterunaAssembly: Alteruna.Trinity (in Alteruna.Trinity.dll) Version: 1.3.4+23572bbe79d00cde564b217419b1eba432b8e858
[SerializableAttribute]
public class SyncedKey
- Inheritance
- Object SyncedKey
Setup to sync the A key and listen to related event.
You can also just as easy get the value directly from the
SyncedKey.Value.
using UnityEngine;
using UnityEngine.Events;
using Alteruna;
public class MyInputClass : MonoBehaviour
{
// Reference to a InputSynchronizable.
public InputSynchronizable Input;
// Key field.
private SyncedKey _myKey;
void Awake()
{
// Setup key.
_myKey = new SyncedKey(Input, KeyCode.A);
// Listen to key event.
_myKey.OnInputChanged.AddListener(KeyChange);
}
void KeyChange(SyncedKey key) {
// This is the same value as _myKey.Value.
Debug.Log(key.Value);
}
}
SyncedKeys can also be set up by the inspector. but to work, they still need to be registered.
using UnityEngine;
using Alteruna;
public class MyJumpClass : MonoBehaviour
{
// Reference to a InputSynchronizable.
public InputSynchronizable Input;
// Jump input that we can setup in the inspector.
public SyncedKey Jump;
// Jump force.
public float jumpForce = 10f;
void Awake()
{
Jump.Register(Input);
}
private void Update()
{
if (Jump)
{
transform.Translate(0, Time.deltaTime * jumpForce, 0);
}
}
}
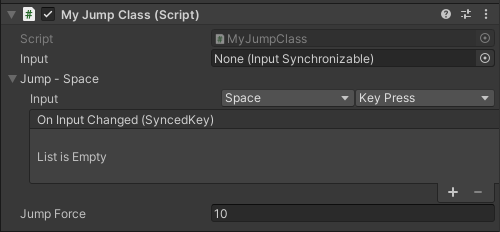
Key |
Registered Keycode input.
On set, reregister if already registered.
|
Value |
Value of target input key.
|