SyncedAxis Class
Alternative way of implementing InputSynchronizable.
Namespace: AlterunaAssembly: Alteruna.Trinity (in Alteruna.Trinity.dll) Version: 1.3.4+03e8eebec78141d9d3b2022fda2c0ac58d3116b9
[SerializableAttribute]
public class SyncedAxis
- Inheritance
- Object SyncedAxis
We can setup a SyncedAxis in the inspector and register it in the Awake method.
Setup to sync the Horizontal axis and use its value.
using Alteruna;
using UnityEngine;
[RequireComponent(typeof(InputSynchronizable))]
public class InputTest : MonoBehaviour
{
public float Speed = 5;
public SyncedAxis AxisX = new SyncedAxis("Horizontal");
public SyncedAxis AxisY = new SyncedAxis("Vertical");
private InputSynchronizable _input;
void Awake()
{
if (_input == null)
_input = GetComponent<InputSynchronizable>();
AxisX.Register(_input);
AxisY.Register(_input);
}
void FixedUpdate()
{
float scaledSpeed = Speed * Time.deltaTime;
transform.Translate(
scaledSpeed * AxisX.Value,
scaledSpeed * AxisY.Value,
0);
}
private void Reset()
{
if (_input == null)
_input = GetComponent<InputSynchronizable>();
}
}
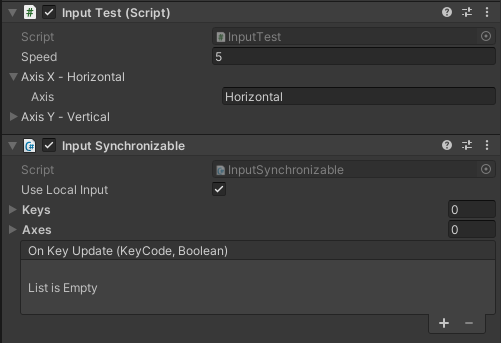